Server Socket Program sample in VB.net
By: Issac in VB.net Tutorials on 2009-02-21
The Server Socket Program is a VB.NET Console based Application. This program acts as a Server and listens to client's request. We have to assign Port 8888 for the Server Socket, it is an instance of the VB.NET Class TcpListener, and call its start () method.
Dim serverSocket As New TcpListener (8888)
serverSocket.Start ()
Next we are creating an infinite loop for continuous communication to Client. When Server gets the request, it reads the data from NetworkStream and also writes the response to NetworkStream. For doing this create a new VB.NET Console Application Project and put the following source code into project.
Imports System.Net.Sockets Imports System.Text Module Module1 Sub Main() Dim serverSocket As New TcpListener(8888) Dim requestCount As Integer Dim clientSocket As TcpClient serverSocket.Start() msg("Server Started") clientSocket = serverSocket.AcceptTcpClient() msg("Accept connection from client") requestCount = 0 While (True) Try requestCount = requestCount + 1 Dim networkStream As NetworkStream = _ clientSocket.GetStream() Dim bytesFrom(10024) As Byte networkStream.Read(bytesFrom, 0, CInt(clientSocket.ReceiveBufferSize)) Dim dataFromClient As String = _ System.Text.Encoding.ASCII.GetString(bytesFrom) dataFromClient = _ dataFromClient.Substring(0, dataFromClient.IndexOf("$")) msg("Data from client - " + dataFromClient) Dim serverResponse As String = _ "Server response " + Convert.ToString(requestCount) Dim sendBytes As [Byte]() = _ Encoding.ASCII.GetBytes(serverResponse) networkStream.Write(sendBytes, 0, sendBytes.Length) networkStream.Flush() msg(serverResponse) Catch ex As Exception MsgBox(ex.ToString) End Try End While clientSocket.Close() serverSocket.Stop() msg("exit") Console.ReadLine() End Sub Sub msg(ByVal mesg As String) mesg.Trim() Console.WriteLine(" >> " + mesg) End Sub End Module
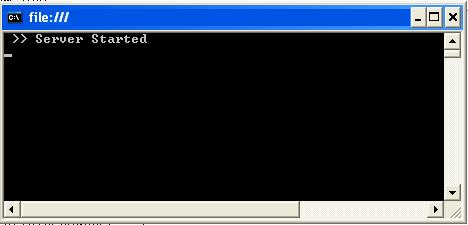
This server socket is in only a part of the socket program,we need a client to test it
fully, we will see about it in detail in the client
socket programming tutorial, you should first start the Server Socket Program and then start the Client Socket Program.
Add Comment
This policy contains information about your privacy. By posting, you are declaring that you understand this policy:
- Your name, rating, website address, town, country, state and comment will be publicly displayed if entered.
- Aside from the data entered into these form fields, other stored data about your comment will include:
- Your IP address (not displayed)
- The time/date of your submission (displayed)
- Your email address will not be shared. It is collected for only two reasons:
- Administrative purposes, should a need to contact you arise.
- To inform you of new comments, should you subscribe to receive notifications.
- A cookie may be set on your computer. This is used to remember your inputs. It will expire by itself.
This policy is subject to change at any time and without notice.
These terms and conditions contain rules about posting comments. By submitting a comment, you are declaring that you agree with these rules:
- Although the administrator will attempt to moderate comments, it is impossible for every comment to have been moderated at any given time.
- You acknowledge that all comments express the views and opinions of the original author and not those of the administrator.
- You agree not to post any material which is knowingly false, obscene, hateful, threatening, harassing or invasive of a person's privacy.
- The administrator has the right to edit, move or remove any comment for any reason and without notice.
Failure to comply with these rules may result in being banned from submitting further comments.
These terms and conditions are subject to change at any time and without notice.
- Data Science
- Android
- React Native
- AJAX
- ASP.net
- C
- C++
- C#
- Cocoa
- Cloud Computing
- HTML5
- Java
- Javascript
- JSF
- JSP
- J2ME
- Java Beans
- EJB
- JDBC
- Linux
- Mac OS X
- iPhone
- MySQL
- Office 365
- Perl
- PHP
- Python
- Ruby
- VB.net
- Hibernate
- Struts
- SAP
- Trends
- Tech Reviews
- WebServices
- XML
- Certification
- Interview
categories
Related Tutorials
Changes in Controls from VB6 to VB.net
Unstructured Exception Handling in VB.net
Structured Exception Handling in VB.net
Creating Sub Procedures in VB.net
Passing a Variable Number of Arguments to Procedures in VB.net
Specifying Optional Arguments with default values in Procedures in VB.net
Preserving a Variable's Values between Procedure Calls in VB.net
Throwing an Exception in VB.net
Comments