How to create an XML file in VB.net
By: Issac in VB.net Tutorials on 2009-02-21
XML is a platform independent language, so if we create an XML file in one platform it can be used in other platforms also.For creating a new XML file in VB.NET, we are using XmlTextWriter class. The class takes FileName and Encoding as arguments. Also we are here passing formating details. The following source code creating an XML file product.xml and add four rows in the file.
Imports System.Xml Public Class Form1 Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim writer As New XmlTextWriter("product.xml", System.Text.Encoding.UTF8) writer.WriteStartDocument(True) writer.Formatting = Formatting.Indented writer.Indentation = 2 writer.WriteStartElement("Table") createNode(1, "Product 1", "1000", writer) createNode(2, "Product 2", "2000", writer) createNode(3, "Product 3", "3000", writer) createNode(4, "Product 4", "4000", writer) writer.WriteEndElement() writer.WriteEndDocument() writer.Close() End Sub Private Sub createNode(ByVal pID As String, ByVal pName As String, ByVal pPrice As String, ByVal writer As XmlTextWriter) writer.WriteStartElement("Product") writer.WriteStartElement("Product_id") writer.WriteString(pID) writer.WriteEndElement() writer.WriteStartElement("Product_name") writer.WriteString(pName) writer.WriteEndElement() writer.WriteStartElement("Product_price") writer.WriteString(pPrice) writer.WriteEndElement() writer.WriteEndElement() End Sub End Class
Output of the above source code:
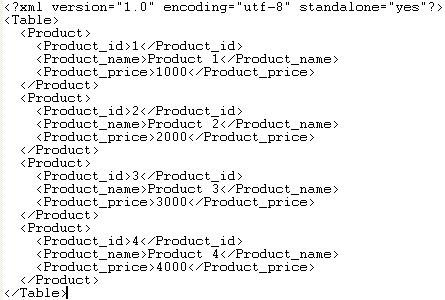
Add Comment
This policy contains information about your privacy. By posting, you are declaring that you understand this policy:
- Your name, rating, website address, town, country, state and comment will be publicly displayed if entered.
- Aside from the data entered into these form fields, other stored data about your comment will include:
- Your IP address (not displayed)
- The time/date of your submission (displayed)
- Your email address will not be shared. It is collected for only two reasons:
- Administrative purposes, should a need to contact you arise.
- To inform you of new comments, should you subscribe to receive notifications.
- A cookie may be set on your computer. This is used to remember your inputs. It will expire by itself.
This policy is subject to change at any time and without notice.
These terms and conditions contain rules about posting comments. By submitting a comment, you are declaring that you agree with these rules:
- Although the administrator will attempt to moderate comments, it is impossible for every comment to have been moderated at any given time.
- You acknowledge that all comments express the views and opinions of the original author and not those of the administrator.
- You agree not to post any material which is knowingly false, obscene, hateful, threatening, harassing or invasive of a person's privacy.
- The administrator has the right to edit, move or remove any comment for any reason and without notice.
Failure to comply with these rules may result in being banned from submitting further comments.
These terms and conditions are subject to change at any time and without notice.
- Data Science
- Android
- React Native
- AJAX
- ASP.net
- C
- C++
- C#
- Cocoa
- Cloud Computing
- HTML5
- Java
- Javascript
- JSF
- JSP
- J2ME
- Java Beans
- EJB
- JDBC
- Linux
- Mac OS X
- iPhone
- MySQL
- Office 365
- Perl
- PHP
- Python
- Ruby
- VB.net
- Hibernate
- Struts
- SAP
- Trends
- Tech Reviews
- WebServices
- XML
- Certification
- Interview
categories
Related Tutorials
Changes in Controls from VB6 to VB.net
Unstructured Exception Handling in VB.net
Structured Exception Handling in VB.net
Creating Sub Procedures in VB.net
Passing a Variable Number of Arguments to Procedures in VB.net
Specifying Optional Arguments with default values in Procedures in VB.net
Preserving a Variable's Values between Procedure Calls in VB.net
Throwing an Exception in VB.net
Comments